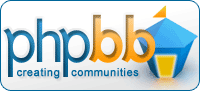 |
dev.gamez.lv Latvian Game Developers Community
|
View previous topic :: View next topic |
Author |
Message |
coderpp

Joined: 20 Aug 2005 Posts: 167 Location: Rīga, Koknese, Gajiena
|
Posted: Sun Nov 13, 2005 1:06 pm Post subject: Vajag palidzibu |
|
Code: | #include <vcl\vcl.h>
#pragma hdrstop
//---------------------------------------------------------------------------
USEFORM("MainWindow.cpp", Form1);
USERES("Breakout.res");
USEUNIT("Ball.cpp");
USEUNIT("Paddle.cpp");
USEUNIT("Block.cpp");
USEFILE("Ball.h");
//---------------------------------------------------------------------------
WINAPI WinMain(HINSTANCE, HINSTANCE, LPSTR, int)
{
try
{
Application->Initialize();
Application->Title = "Simple Breakout";
Application->CreateForm(__classid(TForm1), &Form1);
Application->Run();
}
catch (Exception &exception)
{
Application->ShowException(&exception);
}
return 0;
}
//---------------------------------------------------------------------------
#include <vcl\vcl.h>
#pragma hdrstop
#include "MainWindow.h"
#include <mmsystem.h>
//---------------------------------------------------------------------------
#pragma link "SuperTimer"
#pragma resource "*.dfm"
TForm1 *Form1;
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
width=PaintBox1->Width;
height=PaintBox1->Height;
//Create Memory Bitmap
memBMP=new Graphics::TBitmap();
memBMP->Width=width;
memBMP->Height=height;
//Create new Font objects
font1=new TFont();
font2=new TFont();
ball.paddle=&paddle;
InitializeBlocks();
randomize();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::DrawStuff()
{
//Repaint Background
memBMP->Canvas->Pen->Color=(TColor)RGB(0,0,80);
memBMP->Canvas->Brush->Color=(TColor)RGB(0,0,80);
memBMP->Canvas->Rectangle(0,0,width,height);
if(ball.launched)
ball.Move();
else
ball.x=paddle.x+24;
DrawBlocks();
//Draw Ball
memBMP->Canvas->Pen->Color=(TColor)RGB(255,255,0);
memBMP->Canvas->Brush->Color=(TColor)RGB(255,255,0);
memBMP->Canvas->Ellipse(ball.x,ball.y,ball.x+10,ball.y+10);
if(ball.y>height-10)
{
ball.y=260;
ball.launched=false;
lostBalls++;
PlaySound("ball_lost.wav", NULL, SND_ASYNC | SND_FILENAME);
}
for(i=0;i<23;i++)
{
if(block[i].HitTest())
{
ball.hitBlock=true;
block[i].exist=false;
points++;
PlaySound("click.wav", NULL, SND_ASYNC | SND_FILENAME);
}
}
if(points==23&&ball.launched)
{
points=0;
lostBalls=0;
for(i=0;i<23;i++)
block[i].exist=true;
ball.y=260;
ball.launched=false;
PlaySound("victory.wav", NULL, SND_ASYNC | SND_FILENAME);
}
//Draw Paddle
memBMP->Canvas->Pen->Color=(TColor)RGB(255,50,50);
memBMP->Canvas->Brush->Color=(TColor)RGB(255,50,50);
memBMP->Canvas->RoundRect(paddle.x,270,paddle.x+paddle.width,280,8,8);
DrawText();
//Copy Memory Bitmap to Screen
PaintBox1->Canvas->Draw(0, 0, memBMP);
}
//---------------------------------------------------------------------------
void __fastcall TForm1::DrawText()
{
memBMP->Canvas->Brush->Style = bsClear;
if(!clicked)
{
memBMP->Canvas->Font->Name="Comic Sans MS";
memBMP->Canvas->Font->Size=15;
memBMP->Canvas->Font->Color=clBlue;
memBMP->Canvas->TextOut(47, 122, "Click to launch the ball!");
memBMP->Canvas->Font->Color=clWhite;
memBMP->Canvas->TextOut(45, 120, "Click to launch the ball!");
}
memBMP->Canvas->Font->Name="Arial";
memBMP->Canvas->Font->Size=8;
String blockS;
if(points==1)blockS=" block ";
else blockS=" blocks ";
memBMP->Canvas->Font->Color=clBlue;
memBMP->Canvas->TextOut(11, 283, IntToStr(points)+blockS+"destroyed");
memBMP->Canvas->Font->Color=clWhite;
memBMP->Canvas->TextOut(10, 282, IntToStr(points)+blockS+"destroyed");
String lostS;
if(lostBalls==1)lostS=" ball ";
else lostS=" balls ";
memBMP->Canvas->Font->Color=clBlue;
memBMP->Canvas->TextOut(235, 283, IntToStr(lostBalls)+lostS+"lost");
memBMP->Canvas->Font->Color=clWhite;
memBMP->Canvas->TextOut(234, 282, IntToStr(lostBalls)+lostS+"lost");
}
//---------------------------------------------------------------------------
void __fastcall TForm1::SuperTimer1Timer(TObject *Sender)
{
DrawStuff();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::PaintBox1MouseMove(TObject *Sender, TShiftState Shift,
int X, int Y)
{
paddle.Move(X-26);
}
//---------------------------------------------------------------------------
void __fastcall TForm1::InitializeBlocks()
{
for(i=0;i<23;i++)
block[i].ball=&ball;
for(i=0; i<5; i++)
{
block[i].y=10;
block[i].color=(TColor)RGB(140,140,255);
block[i].x=i*60+15;
block[i+9].y=40;
block[i+9].color=(TColor)RGB(80,80,255);
block[i+9].x=i*60+15;
block[i+18].y=70;
block[i+18].color=(TColor)RGB(20,20,255);
block[i+18].x=i*60+15;
}
for(i=0; i<4; i++)
{
block[i+5].y=25;
block[i+5].color=(TColor)RGB(110,110,255);
block[i+5].x=i*60+45;
block[i+14].y=55;
block[i+14].color=(TColor)RGB(50,50,255);
block[i+14].x=i*60+45;
}
}
void __fastcall TForm1::DrawBlocks()
{
for(i=0;i<5;i++)
{
memBMP->Canvas->Pen->Color=block[i].color;
memBMP->Canvas->Brush->Color=block[i].color;
if(block[i].exist)
memBMP->Canvas->Rectangle(block[i].x, block[i].y, block[i].x+block[i].width, block[i].y+8);
}
for(i=5;i<9;i++)
{
memBMP->Canvas->Pen->Color=block[i].color;
memBMP->Canvas->Brush->Color=block[i].color;
if(block[i].exist)
memBMP->Canvas->Rectangle(block[i].x, block[i].y, block[i].x+block[i].width, block[i].y+8);
}
for(i=9;i<14;i++)
{
memBMP->Canvas->Pen->Color=block[i].color;
memBMP->Canvas->Brush->Color=block[i].color;
if(block[i].exist)
memBMP->Canvas->Rectangle(block[i].x, block[i].y, block[i].x+block[i].width, block[i].y+8);
}
for(i=14;i<18;i++)
{
memBMP->Canvas->Pen->Color=block[i].color;
memBMP->Canvas->Brush->Color=block[i].color;
if(block[i].exist)
memBMP->Canvas->Rectangle(block[i].x, block[i].y, block[i].x+block[i].width, block[i].y+8);
}
for(i=18;i<23;i++)
{
memBMP->Canvas->Pen->Color=block[i].color;
memBMP->Canvas->Brush->Color=block[i].color;
if(block[i].exist)
memBMP->Canvas->Rectangle(block[i].x, block[i].y, block[i].x+block[i].width, block[i].y+8);
}
}
void __fastcall TForm1::FormKeyDown(TObject *Sender, WORD &Key,
TShiftState Shift)
{
if(Key==VK_RIGHT)
{
paddle.Move(paddle.x+=10);
}
else if(Key==VK_LEFT)
{
paddle.Move(paddle.x-=10);
}
}
//---------------------------------------------------------------------------
void __fastcall TForm1::PaintBox1Click(TObject *Sender)
{
clicked=true;
if(ball.launched==false)
PlaySound("launch.wav", NULL, SND_ASYNC | SND_FILENAME);
ball.launched=true;
}
//---------------------------------------------------------------------------
[code]//---------------------------------------------------------------------------
#ifndef MainWindowH
#define MainWindowH
//---------------------------------------------------------------------------
#include <vcl\Classes.hpp>
#include <vcl\Controls.hpp>
#include <vcl\StdCtrls.hpp>
#include <vcl\Forms.hpp>
#include "SuperTimer.hpp"
#include <vcl\ExtCtrls.hpp>
#include "Ball.h"
#include "Paddle.h"
#include "Block.h"
//---------------------------------------------------------------------------
class TForm1 : public TForm
{
__published: // IDE-verwaltete Komponenten
TSuperTimer *SuperTimer1;
TPaintBox *PaintBox1;
void __fastcall SuperTimer1Timer(TObject *Sender);
void __fastcall PaintBox1MouseMove(TObject *Sender, TShiftState Shift, int X,
int Y);
void __fastcall FormKeyDown(TObject *Sender, WORD &Key, TShiftState Shift);
void __fastcall PaintBox1Click(TObject *Sender);
private: // Benutzer-Deklarationen
TFont *font1, *font2;
Graphics::TBitmap *memBMP;
int width, height, points, lostBalls;
TBall ball;
TPaddle paddle;
TBlock block[23];
int i,j;
bool clicked;
void __fastcall TForm1::DrawStuff();
void __fastcall TForm1::InitializeBlocks();
void __fastcall TForm1::DrawBlocks();
void __fastcall TForm1::DrawText();
public: // Benutzer-Deklarationen
__fastcall TForm1(TComponent* Owner);
};
//---------------------------------------------------------------------------
extern TForm1 *Form1;
//---------------------------------------------------------------------------
#endif[/code] |
seit ir tris faili. Vai kaads nevar pateikt ka lai dabuj to pasu rezultatu kas ir tajos failos ar standarta headera failiem? |
|
Back to top |
|
 |
bubu Indago Uzvarētājs

Joined: 23 Mar 2004 Posts: 3223 Location: Riga
|
Posted: Sun Nov 13, 2005 1:57 pm Post subject: |
|
Kas ir standarta headera faili tavā izpratnē? |
|
Back to top |
|
 |
coderpp

Joined: 20 Aug 2005 Posts: 167 Location: Rīga, Koknese, Gajiena
|
Posted: Mon Nov 14, 2005 7:41 am Post subject: |
|
Prieks manis standarta headera faili ir tie kuri nak lidzi programai. Es stradajo ar Dev C++ |
|
Back to top |
|
 |
bubu Indago Uzvarētājs

Joined: 23 Mar 2004 Posts: 3223 Location: Riga
|
Posted: Mon Nov 14, 2005 9:00 am Post subject: |
|
Ko nozīmē - nāk līdzi programmai? Takš dažādiem kompilētājiem var atšķirites header faili, kas nāk līdzi. Vai nu raksti kodu ar tiem, kas nāk tavam izvēlētajam kompilētājam, vai ņem citu kompilētāju.
Manuprāt tas kods, ko tu te iekopēji paredzēts Borland C++ Builder, nevis GCC. Tā ka nepareizo kompilētāju esi paņēmis. |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|